Display Prime Benefit Cards for Signed-In Prime Members
Buy with Prime API is now available for early access
Sign up for early access to the Buy with Prime API using the 'Sign Up' button below. The API may change as Amazon receives feedback and iterates on it.
This topic provides examples of how to render Prime benefit cards by using the Buy with Prime UI library. For all of these examples, the item is offered through Buy with Prime, and the shopper is signed in.
To learn how to use the UI library to incorporate Buy with Prime into your checkout flow, see Steps to Use the UI Library.
For the Buy with Prime UI Library Reference, see Buy with Prime UI Library Reference.
Sample data
Some examples in this topic use the following sample data.
Sample Buyability
const buyability = {
status: "BUYABLE",
}
Sample Reversal Offer Response
const reversalOffers = {
id: "example-reversal-offer-id",
expiresAt: "2024-02-07T19:24:28.572312Z",
reversalItemOffers: [
{
item: {
id: {
__typename: "SkuReversalItemIdentifier",
value: "example-sku-1",
}
},
summary: {
title: "Eligible for Return",
description: "This item can be returned in its original condition for a full refund within 30 days of receipt.",
}
}
]
};
Sample Shopper Buy with Prime Eligibility Response
const shopperBwPEligibility = {
shopperId: "example-shopper-id",
bwpEligibilityStatus: "PRIME",
}
Sample Delivery Preview Response
const deliveryPreview = {
id: "example-delivery-preview-id",
deliveryGroups: [
{id: "example-delivery-group-id",
deliveryOffers: [
{ id: "example-delivery-offer-id",
date: {
earliest: "2023-12-08T04:00:00Z",
latest:"2023-12-08T04:00:00Z"
},
policy:{
messaging: {
messageText: "Tomorrow, Mar 4",
locale: "en-US",
badge: "PRIME"
}
},
"expiresAt":null
}]
}
]
}
Prime benefit cards with delivery estimates
Item is returnable
This example code renders the following image.
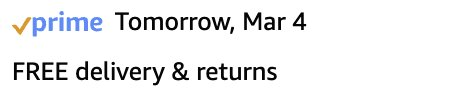
Example Code
// Import the pni-web-components.js in your HTML file
<script type="module" src="/path/to/pni-web-components.js"></script>
//...Other HTML
//Place the web component where desired
<script>
document.addEventListener('DOMContentLoaded', function() {
const primeBenefitCard = document.createElement('prime-benefit-card');
primeBenefitCard.isShopperPrime = true
primeBenefitCard.isItemBuyable = true;
primeBenefitCard.isItemRetunable = true;
primeBenefitCard.deliveryOffer = [{
message: deliveryPreview.deliveryGroups[0].deliveryOffers[0].policy.messaging.messageText,
errorCode: null
}];
document.body.appendChild(primeBenefitCard);
});
</script>
// ...Other HTML
// YourReactComponent.jsx
import { PrimeBadgeReact } from "/path/to/pni-react-components.js";
function YourReactComponent() {
return (
<div>
{/* Place the PrimeBadgeReact component where desired, passing the props */}
<PrimeBadgeReact
isShopperPrime=true
isItemBuyable=true
isItemRetunable=true
deliveryOffer = {message: "Tomorrow, Mar 4", errorCode: null}
/>
</div>
);
}
export default YourReactComponent;
Item isn't returnable
This example code renders the following experience.
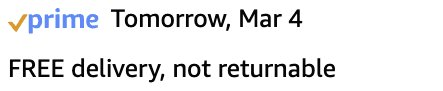
Example Code
<prime-benefit-card id="prime-authenticated-nonReturnable-delivery-offers-badge"></prime-benefit-card>
<script>
const authenticatedNonreturnableWithDeliveryOfferBadge = document.getElementById('prime-authenticated-nonReturnable-delivery-offers-badge');
authenticatedNonreturnableWithDeliveryOfferBadge.deliveryOffer = { message: 'Tomorrow, Mar 4' };
authenticatedNonreturnableWithDeliveryOfferBadge.isShopperPrime = true;
authenticatedNonreturnableWithDeliveryOfferBadge.isItemBuyable = true;
authenticatedNonreturnableWithDeliveryOfferBadge.isItemReturnable = false;
</script>
Delivery estimate is intended but invalid
This example code renders the following experience.

Example Code
<prime-benefit-card id="prime-authenticated-invalid-delivery-offers-badge"></prime-benefit-card>
<script>
const authenticatedWithInvalidAddressBadge = document.getElementById('prime-authenticated-invalid-delivery-offers-badge');
authenticatedWithInvalidAddressBadge.deliveryOffer = { errorCode: 'InvalidDestination' };
authenticatedWithInvalidAddressBadge.isShopperPrime = true;
authenticatedWithInvalidAddressBadge.isItemBuyable = true;
authenticatedWithInvalidAddressBadge.isItemReturnable = true;
</script>
Prime benefit cards without delivery estimates
Item is returnable
This example code renders the following experience.

Example Code
<prime-benefit-card isShopperPrime isItemBuyable isItemReturnable></prime-benefit-card>
Item isn't returnable
This example code renders the following experience.

Example Code
<prime-benefit-card id="prime-authenticated-nonReturnable-badge"></prime-benefit-card>
<script>
const authenticatedNonreturnableBadge = document.getElementById('prime-authenticated-nonReturnable-badge');
authenticatedNonreturnableBadge.isShopperPrime = true;
authenticatedNonreturnableBadge.isItemBuyable = true;
authenticatedNonreturnableBadge.isItemReturnable = false;
</script>
Related topics
Updated 3 days ago